After the huge response and viewership for my earlier article, I have decided to write a new article with all the REST calls example respectively GET, POST, PUT, and DELETE.
Let’s start this project step by step.
Prerequisites for this project:
- If you have Eclipse, download the STS plug-in from here.
- If you don’t have Eclipse, download STS from here.
- Download the latest JDK from here.
- Also for testing please download and install SOAPUI tool from here.
The first example I am going to explain is about HTTP GET request, the second example will be about HTTP POST request, the third example about HTTP PUT request, and the fourth example is for HTTP DELETE request. In all of these examples, I am going to use JSON Representation.
You can download this project from here.
- First, create a folder in your C drive: C:\Projects
- Open eclipse and select work space as: C:\Projects
- From the File menu, select "New" and then "other," and from wizard, expand "Spring Boot" and select "Spring Starter Project" (File->New->other->wizard->Spring Starter Project).
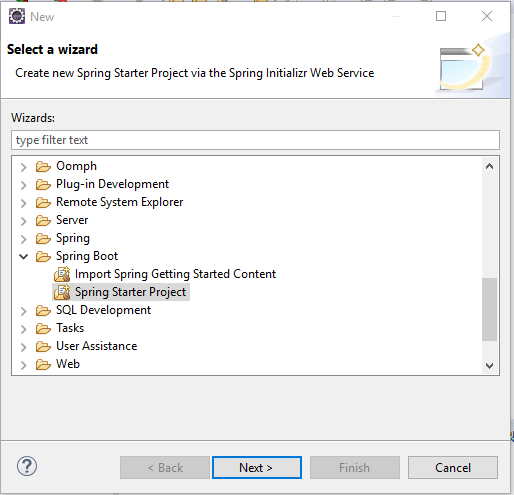
Now click the Next button and provide the below information and click the Next button again.
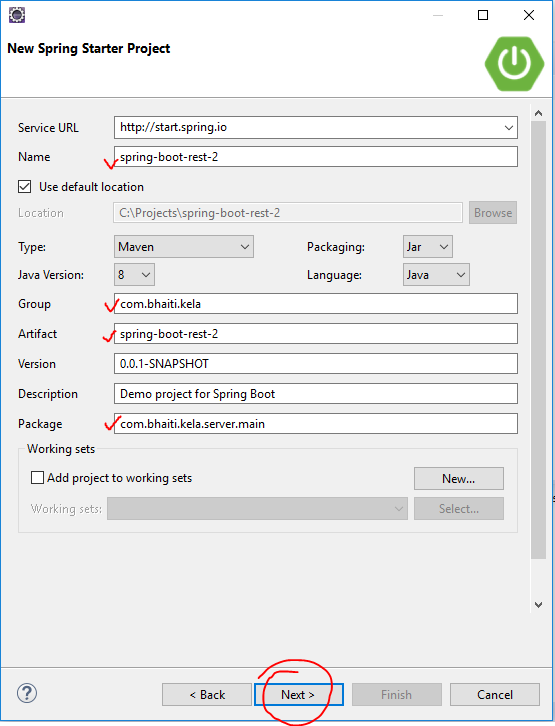
Now, provide/select the below information and click the Finish button.
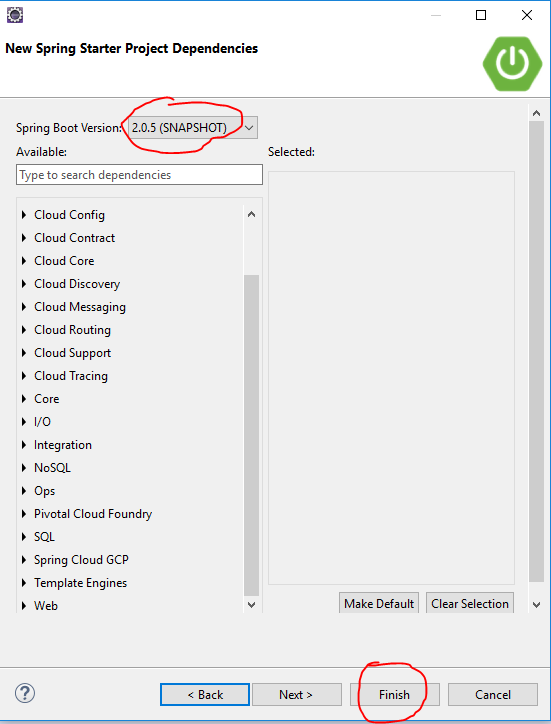
Now you can see the below project structure in your project's explorer window.
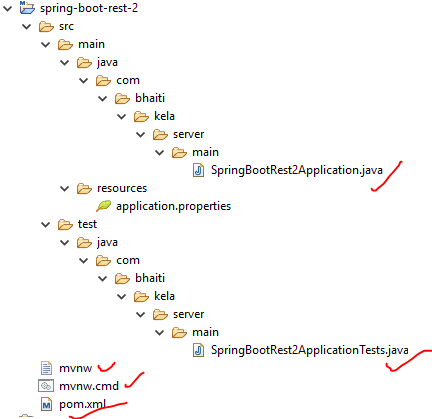
Now, look that the SpringBootRest2Application.java file, which is created by the STS plug-ins.
This is a Spring Boot main class. A Spring Boot REST application loads through this class. We can also see that this class is created with the annotation @SpringBootApplication . As per the Spring documentation, the annotation @SpringBootApplication is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan, and these annotations are frequently used together. Most of the time, in Spring Boot development, the main class is always annotated with all three of these important annotations.
So we are going to modify the @SpringBootApplication (given below in the Java class) with a component path. Without that, the application cannot find out the controller classes. We will learn more about controller classes in a few minutes.
@SpringBootApplication(scanBasePackages = {"com.bhaiti"})
Now we are going to create our beans classes, which we will use for our GET, POST, PUT, and DELETE REST calls. Create package,
com.bhaiti.beans
, and add classes into that package like below:
4. Right-click on the project and select New and then package (New=>Package). Enter the above package name and click Finish.
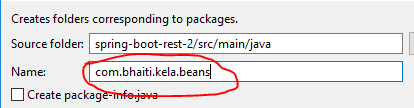
5. Now, right-click on the package
com.bhaiti.beans
and select New->class and provide the class name, Student
, like below: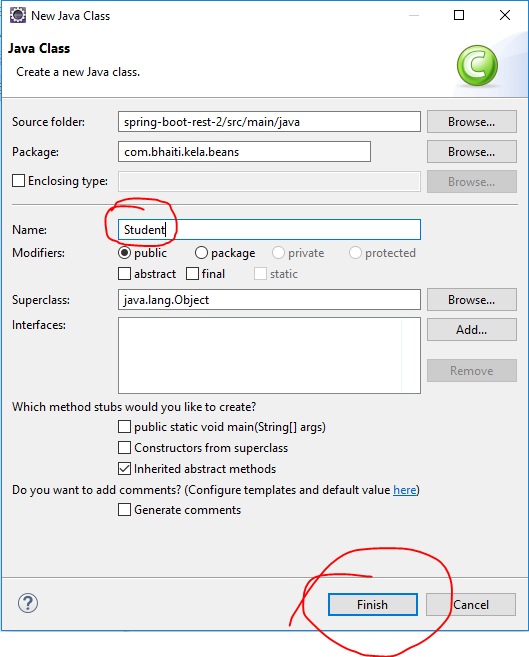
- In the empty class, just add below members:
- Now create getter and setter methods for these members. For that, from the Project Explorer window, right-click on the class "Student" and select “Source” and select “Generate Getter and Setter.”
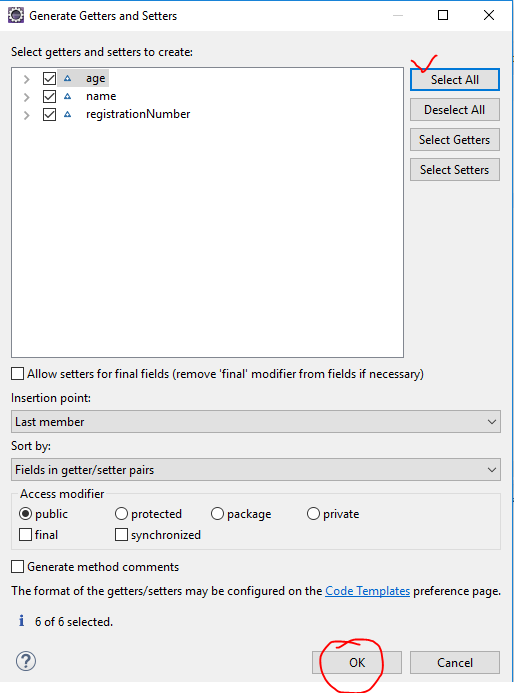
6. Now follow steps 5 and create class call StudentRegistration and modify it like below:
7. Now add a class calls StudentRegistrationReply and modify like below. This class will be used to reply a response back to the client application
8. Now we will introduce two controllers, one to serve the GET request and the second one to serve the POST request. With the GET request, we will retrieve all Student Registration information, and with the POST request, we will add student information into our application. In spring’s approach to build a RESTful web services, HTTP requests are handled by a controller. Controller classes/components are easily identified by the @RestController annotation, and the below StudentRetrieveController will handle GET requests for /student/allstudent by returning a list of Student class objects in JSON format.
- Now just follow step 4 and step 5 and create the package com.bhaiti.kela.controllers and add the class StudentRetrieveController to it and import the class Student and modify the class like below:-
The @RequestMapping annotation maps all HTTP operations by default and, in this example, it ensures that HTTP requests to /student/allstudent are mapped to the getAllStudents() method.
Now we are done with everything for a GET RESTful call. Let’s implement a RESTFul POST call.
- Now it's time to introduce the controller class to handle the POST request. Follow step 5 and add below controller class StudentRegistrationController in package com.bhaiti.kela.controllers
Till now, we are done with everything for a GET and POST RESTful call. Let’s test this application first. After the test, we will learn about PUT and DELETE calls as well. First, we need to compile the application.
- Compilation: To compile this project with Maven, we will add the below information into the POM file: Double click and open the POM file from project explorer window and add below information under “<dependencies>” section.
And we'll also add the below information for the property file for this project under the build section of our POM.xml file (to change the port number at the moment):
your POM.xml file finally looks like below:
9. Now open file application.properties under C:\Projects\spring-boot-rest-2\src\main\resources and add the below lines in it:
server.port=8083
spring.profiles.active=@spring.profiles.active@
10. Now open the command prompt window and go to the project home directory in the command prompt. In my case, it looks like this:
cd C:\Projects\spring-boot-rest-2
mvnw clean package
If everything goes fine, you can see the below result:
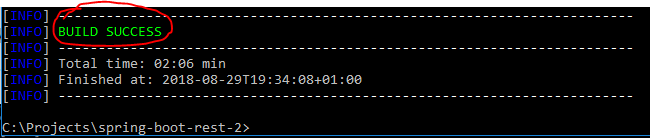
Now run the server:
java -jar target\spring-boot-rest-2-0.0.1-SNAPSHOT.jar

11. Once the server starts successfully, we will test get GET request first. Open your SOAPUI tool. In SOAPUI tool from file menu select New REST Project and put the below URL in the address bar and press OK. (File=>New REST Project)
http://localhost:8083/student/allstudent
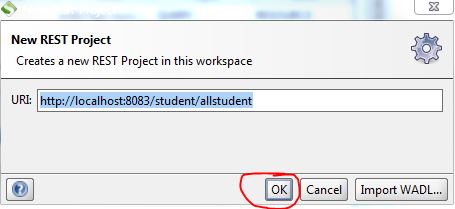
12. Now, from the SOAPUI project explorer menu, expand your project and double click on Request1 and click the green arrow button:
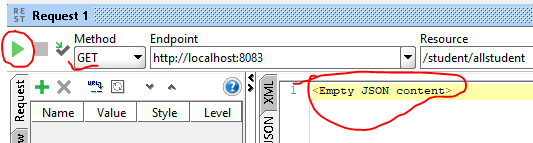
Now you can see the above information. The reason is our student list is empty at the moment, and to store student information, we need to insert some values in it. For that, we will use the POST service that we have already implemented.
13. Follow step 10 and insert the below URL in the address box and click OK.
http://localhost:8083/register/student
14. Now you have to select POST this time from Method combo box for a post request. Once you select POST Media Type, sub pane will emerge from where you have to select media type to application/json like below and put the below json body in it and click the green arrow button, which will add the information into our application.
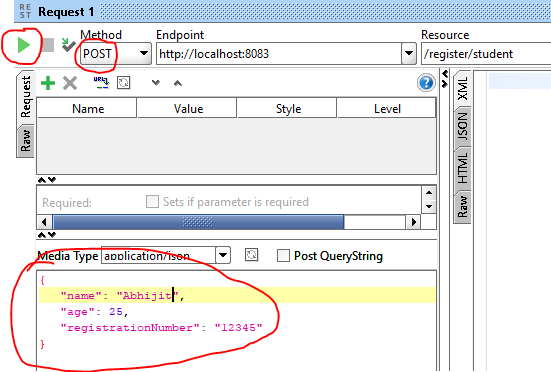
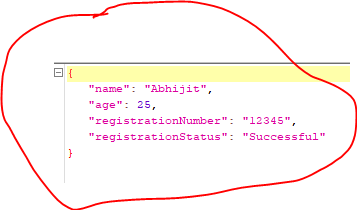
15. Now go to the GET request project (step 12) and click the green arrow button. You can see one record like below:
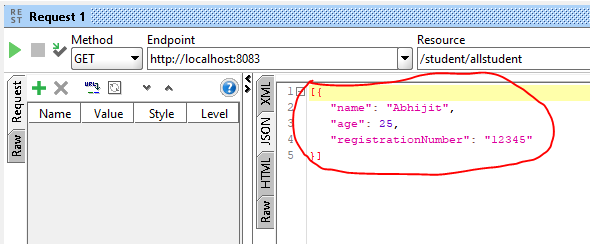
16. Now go back to POST request test (step 14) and add at least three records and call the GET request (step 11) and see:
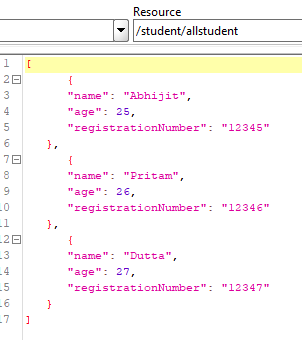
Until now, we have learned how to implement GET and POST services. Now we will learn PUT and DELETE requests.
17. Now introduce the controller classes to handle PUT and DELETE requests. Follow Step 6 above and add the below two controller classes in it.
Create StudentUpdateController class and modify it like below:
Create StudentDeleteController and modify it like below:
NB: In reality, you don’t need four controller classes to handle four different REST service calls. One controller class will suffice, but for clarity, I have introduced four different controller classes in this article.
18. Now stop the server (by using Control-C), and compile the code, and run the server again.
19. First, insert three to four records into the system by using POST call and retrieve the records by using GET test mentioned in step 12.
20. Now we will modify a record here, for that create a new REST project in SOAPUI tool and use the below URL and this time select PUT from method combo box and modify the records as shown below:
http://localhost:8083/update/student
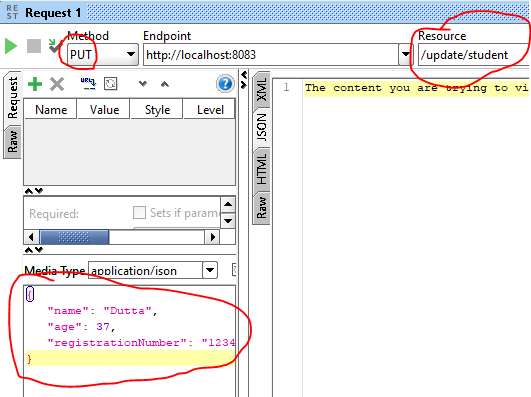
Once you click green arrow button this information will be updated in the system. Now see the result, just do the GET call and check.
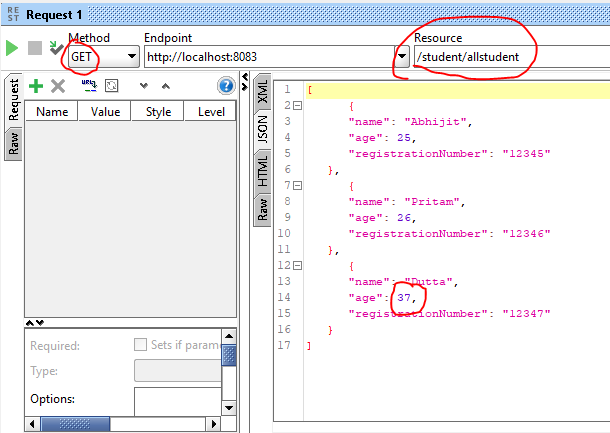
21. Now finally we will do the DELETE service call test. Just create a REST project in SOAPUI and use below URL and select DELETE from method combo box and enter the record like below and click the green arrow button and to check the final outcome just call the GET service call.
http://localhost:8083/delete/student/12346 (the last numeric value is registrationNumber)
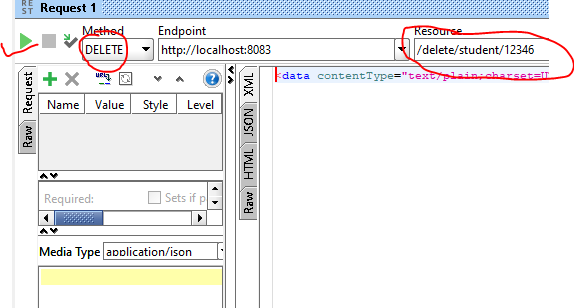
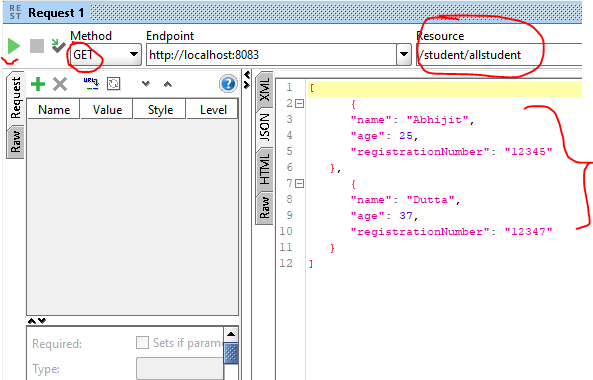
If you liked this article, please do not forget to click the like button and let me know your thoughts in the comments section. Thanks!